What is NPM?
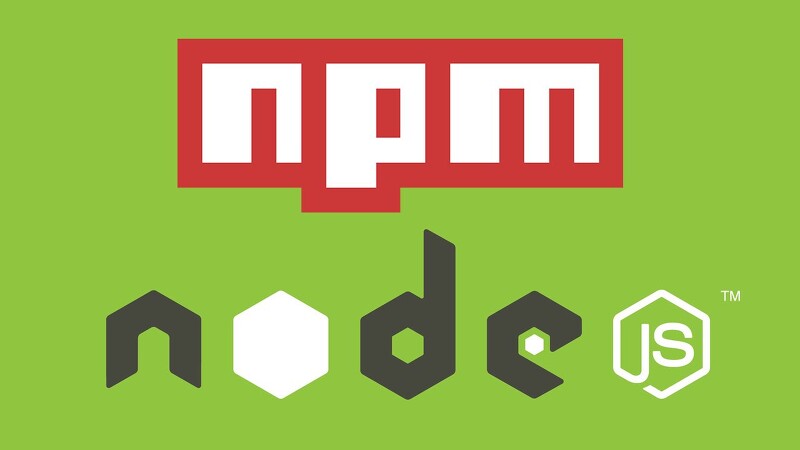
NPM - Node Package Manager is the default package manager for JavaScription's runtime NodeJS.
Having two main parts:
- An online repository for JavaScript packages/modules.
- A command-line interface tool for publishing and downloading packages.
To verify the NPM version, open the command-line interface then enter the script below:
npm --version
What is a package.json file?
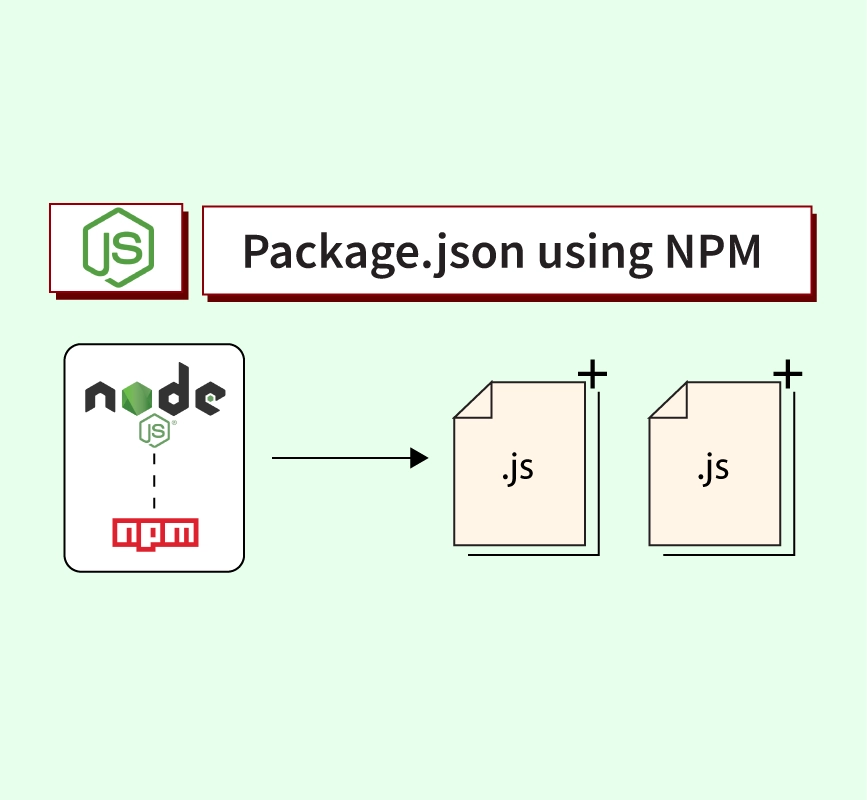
How to create a package.json file? The package.json file will be generated when running the command-line interface below:
npm init
Identifying metadata:
- name: the name of the package/project. Less than 215 characters, have to lowercase, allow hyphens and underscores.
"name": "dinhthanhcong-api"
- version: This is very important when publishing a package/project. Ensure others are using the proper version of a package.
"version": "1.0.0"
- license: This is to limit the use of your package/project by developers or organizations. Value like MIT, ISC, or GPC-3.0
"license": "MIT"
- description: the project's description. This is a short summary of what the package is for.
"description": "API(s) are for dinhthanhcong.info blog"
- keywords: This is indexed by NPM to help find packages. The value is an array of strings.
"keywords": ["API", "blog", "dinhthanhcong"]
Functional metadata:
- main: The entry point into your project, and commonly the file used to start the project.
"main": "dist/index.js"
- repository: This is defined where the source code like Github, Bitbucket,... for open source projects.
"repository": {
"type": "git",
"url": "https://github.com/thanhcong240295"
}
- scripts: Scripts are frequently used to test, build, and streamline the needed commands to work with a package/project.
"scripts": {
"build": "rm -rf ./dist && rm -f tsconfig.tsbuildinfo && npx tsc && tsc-alias -p tsconfig.json",
"start": "pm2 start --env production && pm2 logs -n app-name --raw",
"dev": "nodemon"
}
For example, to start the project base on the dev environment. You will run the command below:
npm run dev
- dependencies: This is one of the most important fields in your package.json. All of the dependencies your project uses are listed here. When a package is installed using the npm CLI, it is downloaded to your node_modules/ folder and an entry is added to your dependencies property, noting the name of the package and the installed version. The carets (^) and tildes (~) you see in the dependency versions are notations for version ranges defined in SemVer.
"dependencies": {
"aws-sdk": "^2.1338.0",
"body-parser": "^1.20.1",
"cors": "^2.8.5",
"dotenv": "^16.0.3"
}
- devDependencies: Similar to the dependencies field, but for packages that are only needed during development, and aren't needed in production.
"devDependencies": {
"@trivago/prettier-plugin-sort-imports": "^4.0.0",
"@types/express": "^4.17.17",
"@types/module-alias": "2.0.1",
"@types/multer": "^1.4.7",
"@types/node": "^18.13.0"
}
What is a package-lock.json file?
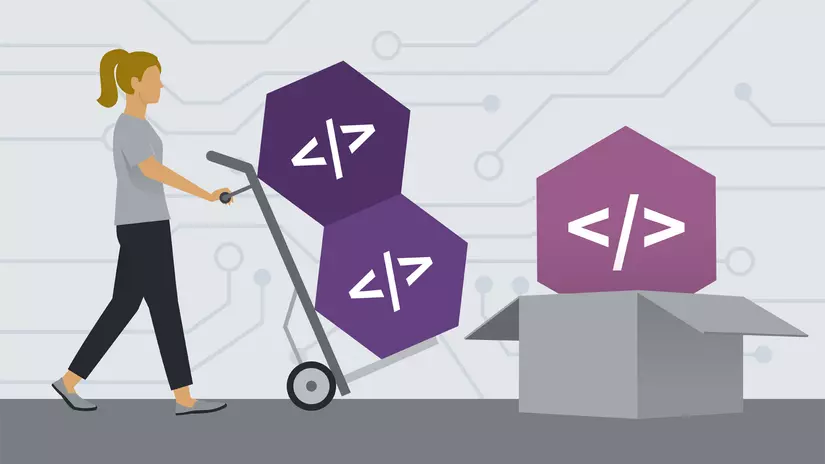
- The package-lock.json file is a snapshot of our entire dependency tree and all the information npm needs to recreate the state of the node_modules/ folder.
- However, The human can see it, the package-lock.json should be not modified manually because the package-lock.json file is generated via the npm CLI.
- It should be committed to version control to ensure the same dependencies on installation.
Note: never commit the node-modules folder. It is not intended to be committed, it's too big, and the state is already tracked.
How to Use Semantic Versioning in NPM
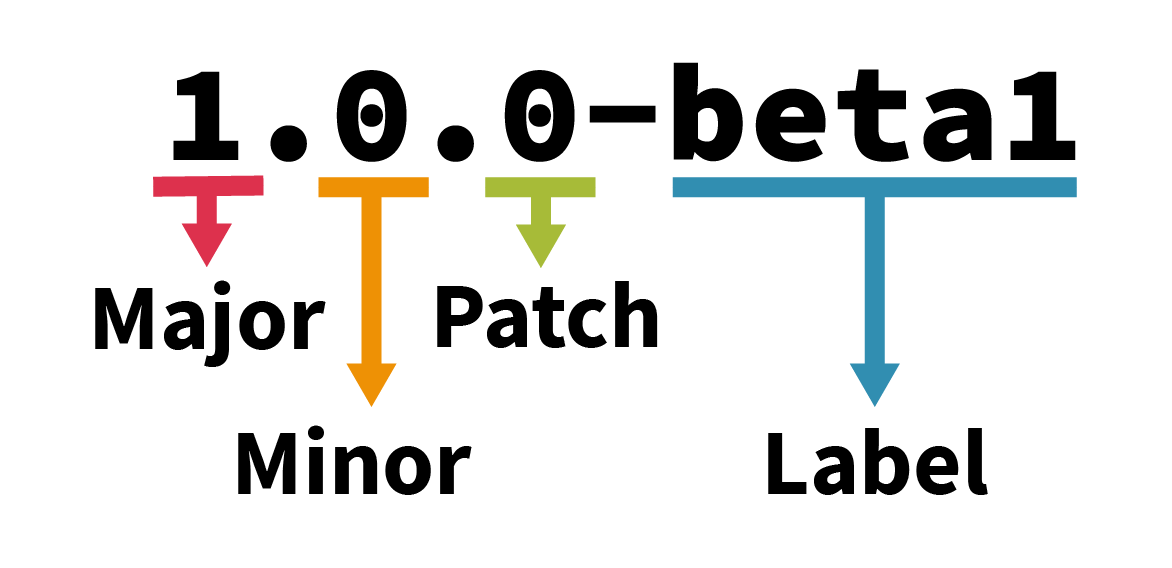
Node packages "GENERALLY" follow semantic versioning. This is just a spec defining three numbers separated by dots, which are:
MAJOR.MINOR.PATCH
Major
- The major version should increase when we’ve introduced new functionality which breaks our API, i.e., increase this value when we’ve added a backward-incompatible change to our project.
- When this number is increased, we must reset the Minor and Patch numbers to 0.
Minor
- We should increase our minor version when we’ve introduced new functionality which changes our API but is backward compatible, i.e., a non-breaking change.
- When we change the Minor version we should also reset the patch version to 0
Patch
- The patch is the number that we increment when we are patching or coding something like a bug or a performance issue.
Version ranges
- If you check your package.json, you will notice that we have symbols all around like tildes (~) and carets (^) next to our semantic version. These symbols are used to define the range of versions that we will be installing.
- The tilde (~) is used to define that we will be installing something that is just a patch, but we will not be going above the current minor version.
- The caret (^) defines that we will be installing anything that is above or minor and patch versions, but never the major because that introduces breaking changes.
Documents
- Tutorialspoint
- Heynode
- Mariokandut
- Baeldung